I hate minutiae... and I'll go to any lengths (of minutiae) to automate things I want to do multiple times. Here is a base script that can be used to place multiple buttons/levers on a wall in rows and columns optionally specifying the wall space to contain them and optionally omitting specific cells. It creates the controls (only tested with wall_button and lever objects) and connects them to a sample routine that can process the input. You'll probably not want to use the defaults, but it's a starting point. It defines the controls in the same square and facing as the LUA script - this can be a little tricky manually to get the rotations and adjustments right especially given setWorldPosition() can cause buttons to recede behind a wall even if the coordinates aren't altered! (Thanks Blichew!)
Format: makeControls(caller, control, base, size, space, omit)
- caller - a required object; indicates the base for the controls; results in coordinates for the controls.
- control - a required string; defines what is to be placed; "wall_button" and "lever" have been tested.
- base - a required string; the base string for all objects created. i.e. self.go.id.."_ctrl1_"
- size - an optional vector; the size of the matrix to be produced. i.e. {3, 3}
- space - an optional vector; the offset wall space the controls will occupy. Buttons work in {1.1, -.7, 1, -1}, levers in {.9, -.6, 1, -1} although you can push them into adjacent squares with higher values. If nil or partial vectors are specified, missing elements will default to these values.
- omit - an optional vector of combined row..col numbers to omit. i.e. {11,22} omits cells 1, 1 and 2, 2.
Code: Select all
makeControls(self, "wall_button", self.go.id .."_button_")
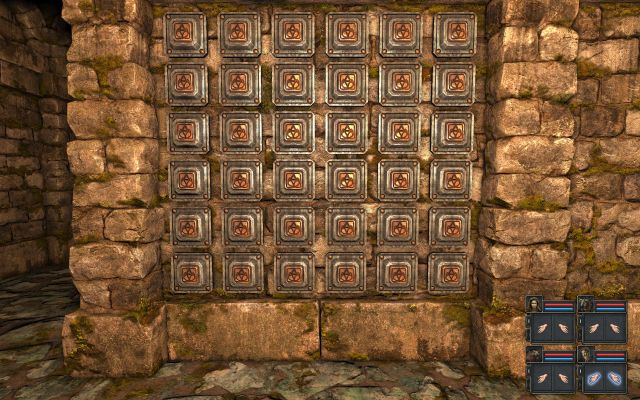
Code: Select all
makeControls(self, "lever", self.go.id .."_lever_")
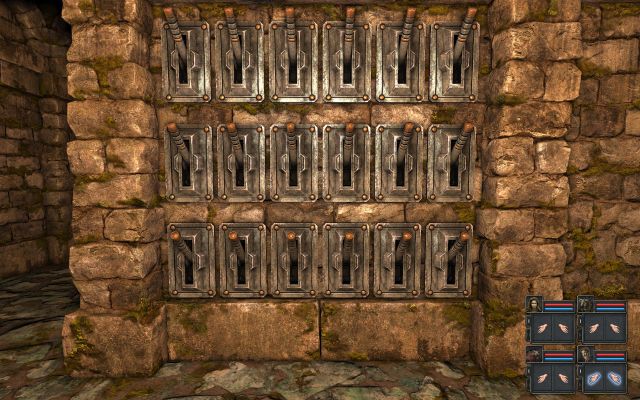
Code: Select all
makeControls(self, "wall_button", self.go.id .. "_button_", {3, 5}, {1, .2, 1, -1}, {14, 15, 24, 25})
makeControls(self, "lever", self.go.id .. "_lever_", {1, 2}, {.86, .6, -.5})
The second call defines 1 row of 2 levers {1, 2} in the offsets {.86, .6, -.5} but note that one offset, the last, is missing. It defaulted to -1 for levers - see space above.
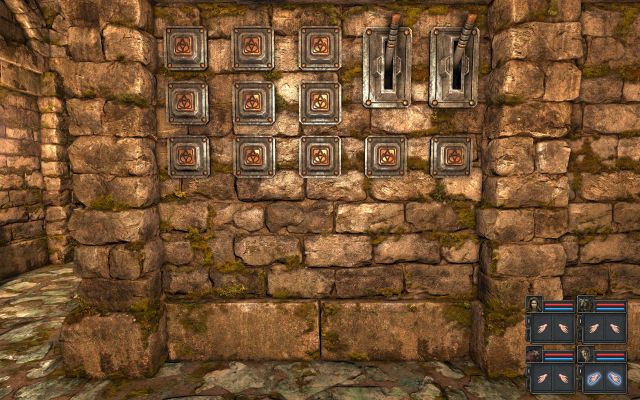
Code: Select all
makeControls(self, "wall_button", self.go.id .."_button_", {3, 3}, {.4, -.4, .4, -.4}, {21, 23, 12, 32})
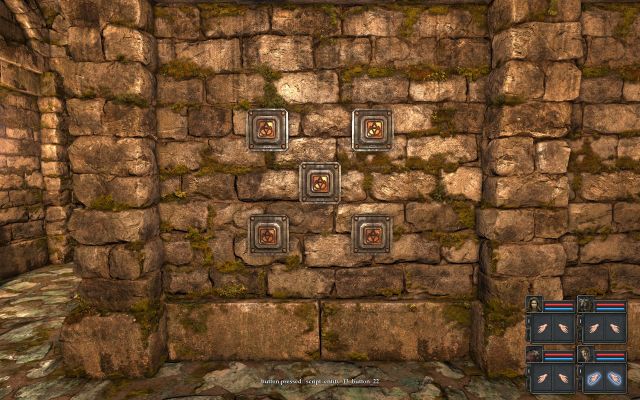
Code: Select all
function makeControls(my, what, base, size, range, omit)
local isLever = what == "lever"
if size == nil then
if what == "lever" then
size = {3, 6}
else
size = {6, 6}
end
end
local area
local rows, cols = size[1], size[2]
if isLever then area = {.9, -.6, 1, -1}
else area = {1.1, -.7, 1, -1}
end
if range ~= nil then
for i = 1, 4 do
if range[i] ~= nil then
area[i] = range[i]
end
end
end
local drop = {}
if omit ~= nil then
for i = 1, # omit do
drop[tostring(omit[i])] = true
end
end
local r1, r2, c1, c2 = area[1], area[2], area[3], area[4]
if rows == 1 then rdiv = 1 else rdiv = rows - 1 end
if cols == 1 then cdiv = 1 else cdiv = cols - 1 end
local dr, dc = (r1 - r2) / rdiv, (c1 - c2) / cdiv
local i, k = self.go.facing % 2 * 2 + 1, (self.go.facing + 1) % 2 * 2 + 1
local ar, ac = getForward(self.go.facing)
if self.go.facing == 1 or self.go.facing == 2 then sign = 1
else sign = -1
end
local adj = 0.0001 * (ar - ac)
for row = 1, rows do
local r = r1 - (row - 1) * dr
for col = 1, cols do
if not drop[row..col] then
local c = c1 - (col - 1) * dc
local ctl = spawn(what, my.go.level, my.go.x, my.go.y, my.go.facing, my.go.elevation, base..row..col)
local vec = ctl:getWorldPosition()
vec[2] = vec[2] + r
vec[i] = vec[i] + c * sign
vec[k] = vec[k] - adj
ctl:setWorldPosition(vec)
if isLever then ctl.lever:addConnector("onToggle", my.go.id, "leverFlip")
else ctl.button:addConnector("onActivate", my.go.id, "buttonPress")
end
end
end
end
end
function buttonPress(my)
local pushed = tonumber(string.sub(my.go.id, string.len(my.go.id) - 1))
hudPrint("button pressed: "..pushed)
--Replace the above with your own logic
end
function leverFlip(my)
local lev = findEntity(my.go.id)
local flipped = tonumber(string.sub(my.go.id, string.len(my.go.id) - 1))
hudPrint("lever flipped: "..flipped.." has been " .. (lev.lever:isActivated() and "activated" or "deactivated"))
--Replace the above with your own logic
end
makeControls(self, "wall_button", self.go.id .."_button_", {3, 3}, {.4, -.4, .4, -.4}, {21, 23, 12, 32})
[EDIT] Updated buttonPress() and leverFlip() examples and added a missing local statement.